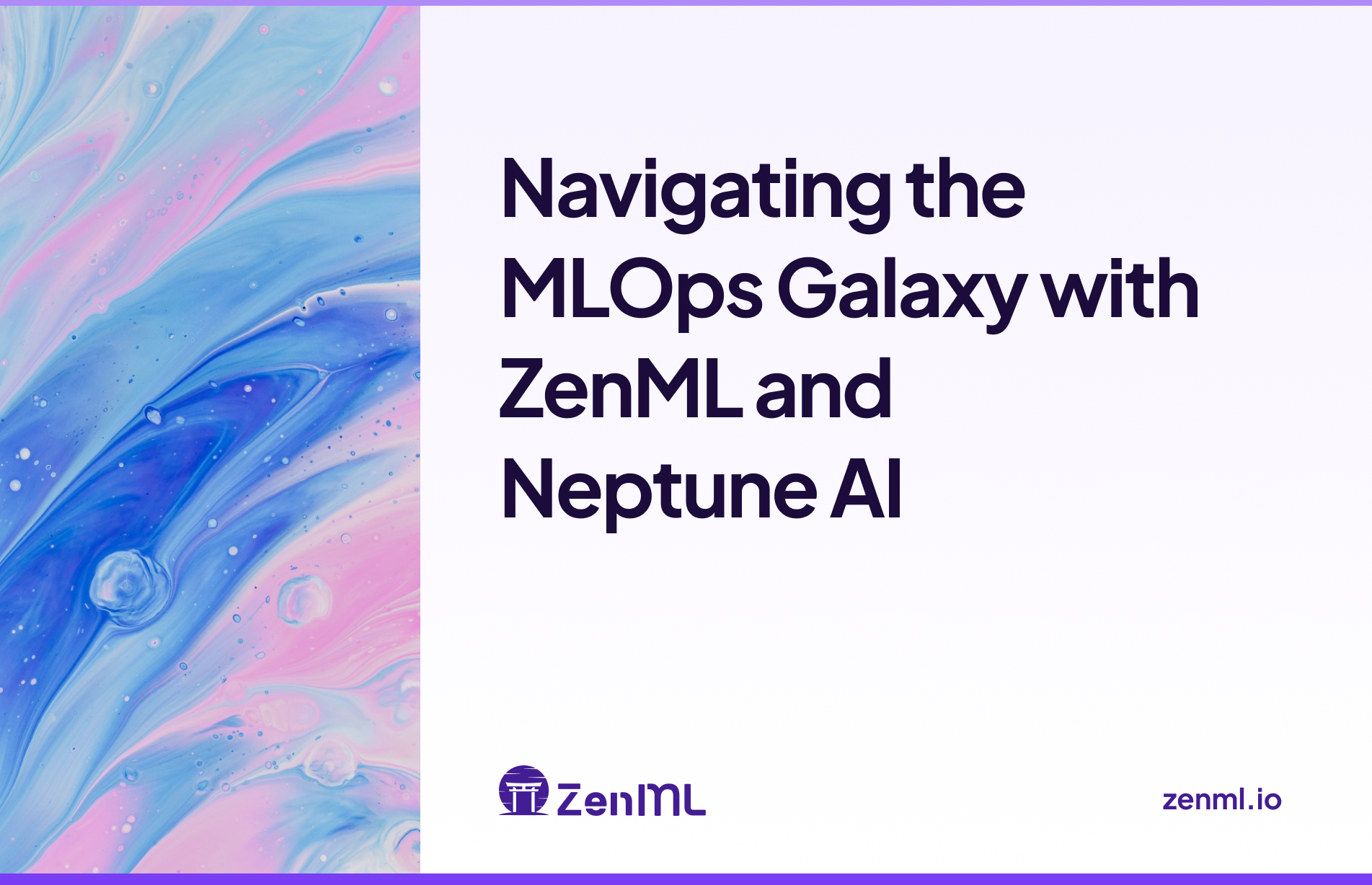
Let's start with a reality check that might feel uncomfortably familiar. In 2024, where does 90% of your model iteration history actually live?
- Jupyter notebooks named "final_v3_REALLY_FINAL.ipynb"
- Random experiment runs with commit hashes that don't exist anymore
- That one CSV your colleague sent over Slack last month
- Your browser history because you forgot to save the tensorboard URL
Sound familiar? You're not alone. While the MLOps ecosystem offers countless tools for experiment tracking, many teams still struggle with these basic challenges. Here's why: We've been treating experiment tracking as a tooling problem when it's fundamentally a workflow problem.
The Three Deadly Sins of ML Experiment Tracking
Before we dive into solutions, let's acknowledge the workflow mistakes that plague even well-equipped teams:
- Running Experiments Before Defining What We're Measuring
- We jump into training without clear success criteria
- Metrics get added as afterthoughts
- Different team members track different metrics
- Not Versioning the Data
- Test sets evolve without documentation
- Benchmark datasets change between experiments
- No clear record of data preprocessing steps
- Assuming We'll "Remember the Important Details"
- Critical hyperparameters go unlogged
- Environment configurations are lost
- Model architecture decisions remain undocumented
A Workflow-First Approach to ML Experiment Tracking
Pre-Experiment Documentation
Before any code is written or models are trained, teams must complete an experiment definition document that includes:
- Primary and secondary metrics with specific thresholds
- Clear definition of what constitutes a "better" model
- Required comparison metrics for A/B testing
- Stakeholder sign-off on success criteria
The key is making this documentation a required gateway - no training runs begin without it. This can be as simple as a shared template that must be filled out, or as robust as a formal approval process.
Data Versioning Protocol
Establish a systematic approach to data management:
- Create a data registry that tracks every version of training and evaluation datasets
- Document all preprocessing steps in a versioned configuration file
- Maintain a changelog for data modifications
- Store fixed evaluation sets with unique identifiers
- Create automated checks that prevent training without data versioning information
The focus here is on making data versioning automatic and mandatory rather than optional.
Experiment Metadata System
Implement a structured logging system that requires:
- Mandatory recording of environment details before experiments start
- Standard templates for hyperparameter documentation
- Automated capture of all model architecture decisions
- Regular experiment summary reports
- Team review sessions to ensure important context is captured
The key innovation here is shifting from "remember to log" to "unable to proceed without logging."
This workflow creates natural stopping points where teams must properly document and version before proceeding, making good practices the path of least resistance rather than an afterthought.
Building a Better Workflow: Using ZenML and Neptune
A practical implementation to the above can be seen with the powerful combination of ZenML and Neptune comes in. We'll explore how integrating these two tools can streamline your ML workflows and provide increased visibility into your experiments.
ZenML is an extensible, open-source MLOps framework designed to create production-ready machine learning pipelines. It offers a simple, intuitive API that allows you to define your ML workflows as a series of steps, making it easy to manage complex pipelines.
Neptune is an experiment tracker built for large-scale model training. It allows AI researchers to monitor their model training in real-time, visualize and compare experiments, and collaborate on them with a team.
When combined, these tools offer a robust solution for managing your entire ML lifecycle, from experimentation to production.
A Real-World Example: Fine-Tuning a Language Model
Let's dive into a practical example of how ZenML and Neptune can work together to enhance your ML workflows. We'll create a pipeline for fine-tuning a language model, tracking the entire process with Neptune.
Setting the Stage: Environment Setup
First, let's get our environment ready:
Next, we'll configure our Neptune credentials using ZenML secrets:
Now, let's register the Neptune experiment tracker in our ZenML stack:
.png)
Architecting the Pipeline
Here's our ZenML pipeline for fine-tuning a DistilBERT model:
This pipeline accomplishes the following:
- Prepares a subset of the IMDB dataset for sentiment analysis.
- Fine-tunes a DistilBERT model on this dataset.
- Evaluates the model and logs the metrics to Neptune.
Launching the Pipeline and Exploring Results
Now, let's set our pipeline in motion:
.png)
As the pipeline runs, ZenML automatically creates Neptune experiments for each step where tracking is enabled. You can view these experiments in the Neptune UI by visiting https://app.neptune.ai/YOUR_WORKSPACE/YOUR_PROJECT/experiments.
.png)
In the Neptune UI, you'll have access to a wealth of information:
- Detailed metrics for your fine-tuning run, including accuracy, F1 score, precision, and recall.
- Comparisons between different runs of your pipeline to identify improvements or regressions.
- Training curves to visualize how your model's performance evolved during training.
- Collaboration tools to share results with team members for joint analysis and decision-making.
.png)
Beyond Tools: Building a Culture of Experiment Tracking
Remember:
- Tools enable good practices; they don't create them
- Start with workflow design, then choose supporting tools
- Create processes that make good practices the path of least resistance
Conclusion: Fix Your Workflow First
While tools like ZenML and Neptune are powerful allies in ML development, they're most effective when supporting well-designed workflows. Before diving into tool selection:
- Define clear tracking requirements
- Establish data versioning protocols
- Create explicit documentation requirements
- Build processes that enforce good practices
The best experiment tracking setup is the one your team will actually use consistently. Start with workflow, and let the tools serve your process - not the other way around.
Ready to improve your ML experiment tracking? Start by examining your workflow, then let tools like ZenML and Neptune help you implement and enforce good practices.